Introduction
In today’s digital age, online quizzes have become a popular way to evaluate and assess knowledge. An online quiz system project in PHP offers numerous advantages over pen-and-paper tests.
So in this blog post, we develop a PHP multiple choice Quiz source code project from scratch. No worry! We guide you through the whole process. We use PHP, a popular server-side scripting language, with a database built around MySQL.
And if you want to learn how to build a simple PHP cart, check out our article here.
We will code a fully functional online quiz system. Complete with a user-friendly interface powered by BootStrap 5. Hooked with MySQL database connectivity. Additionally, we will provide you with the complete source code for the project, which you can use as a basis for your own quiz system. Or customize it to meet your specific needs.
Our guide will help you build a robust and effective online quiz system in PHP. So let’s dive in! Here is a demo:
Establish Database
We can use the GUI tool of phpMyAdmin to provide a user-friendly experience for managing the MySQL database named “quiz”.
In this database, we create 3 main tables named:
- users: here we can track the login of different users.
- questions: in this table, we enter questions for our quiz app.
- answers: in this table, we will record our answers to questions linked with “ans_id”.
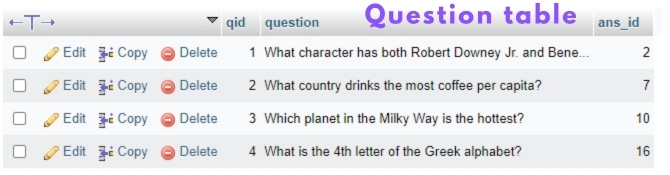
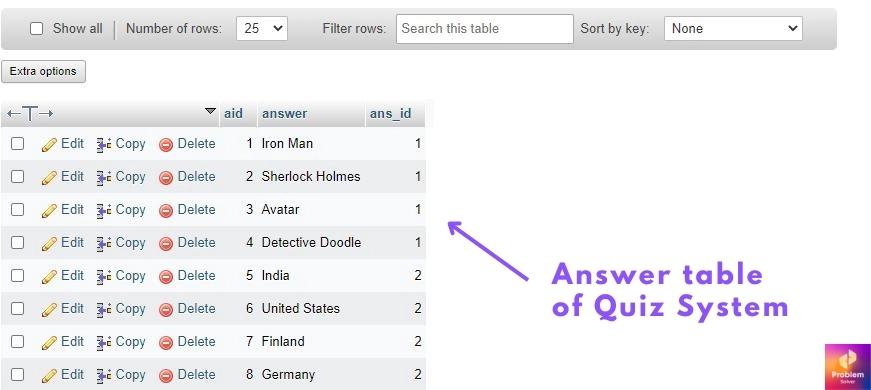
Finally, using the GUI tool of phpMyAdmin provides a user-friendly experience for managing the MySQL database named “quiz”. Also, you can download this database file from the below link:
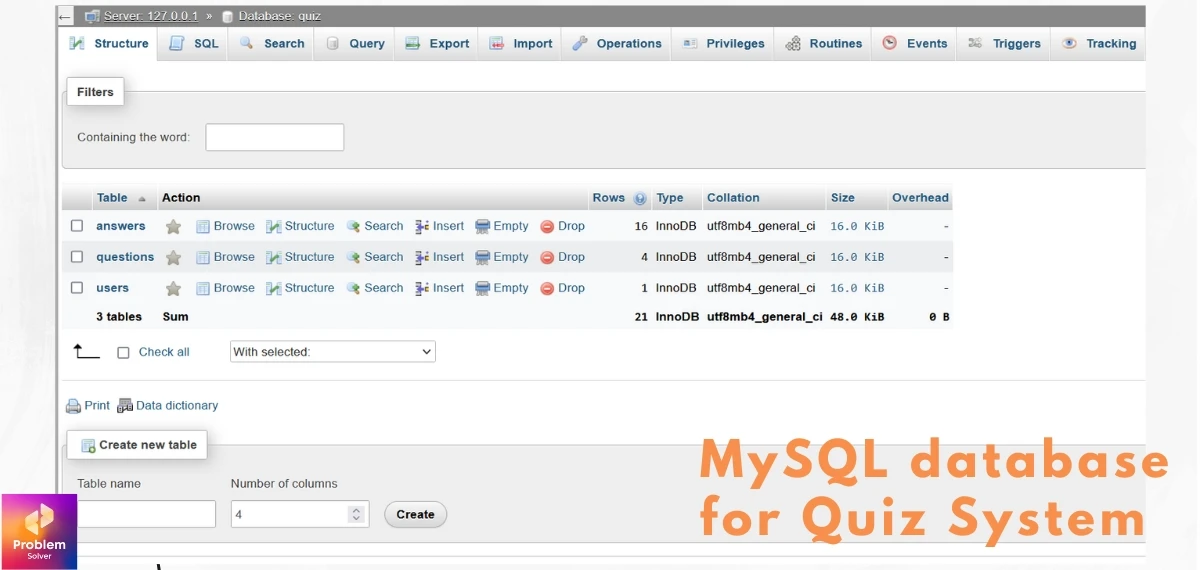
Login and SignUp
Here is the code below for our login page which is an “index.php” file in our case. If you want details on how to follow step by step guide to building a custom login and signup functionality in PHP, then check out this article.
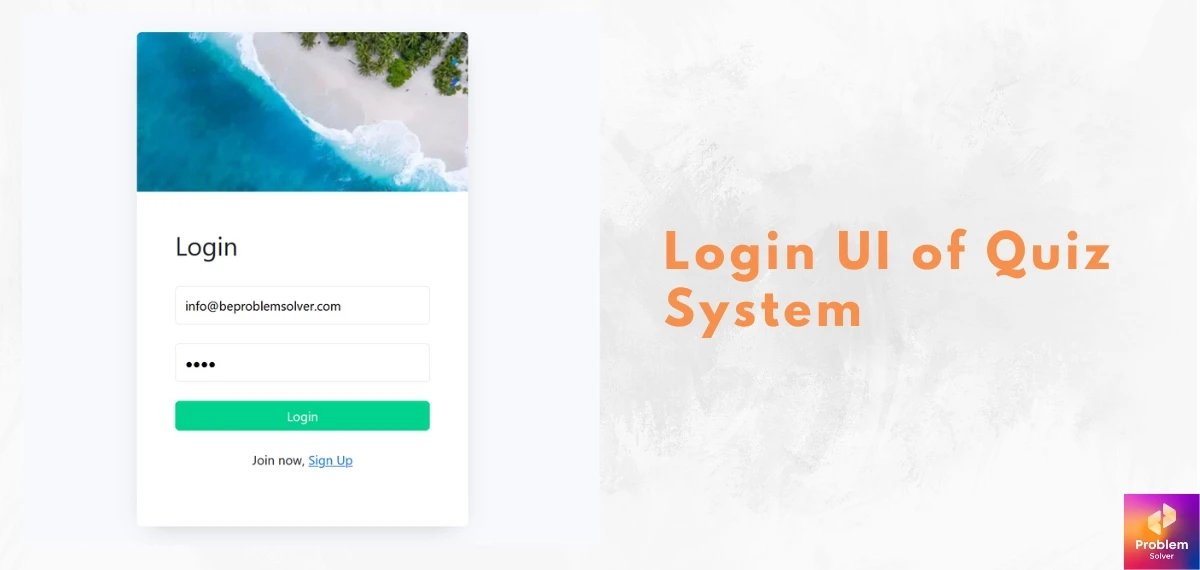
index.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); require_once("function.php"); session_start(); if (isset($_SESSION['login_active'])) { header("Location: dashboard.php"); exit(); } else { if (isset($_POST['login'])) { $email = santize($_POST['email']); $inputpassword = santize($_POST['password']); $password = md5($inputpassword); $sql = "SELECT * FROM users WHERE email = '$email' AND password = '$password'"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { while ($row = mysqli_fetch_assoc($result)) { $_SESSION['login_active'] = [$row["name"], $row["email"]]; $_SESSION['msg'] = "Welcome to Dashboard"; $_SESSION['class'] = "text-bg-success"; header("Location: dashboard.php"); exit(); } } else { $_SESSION['msg'] = "Check Email & Password"; $_SESSION['class'] = "text-bg-danger"; header("Location: index.php"); exit(); } } } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz Login</title> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="css/style.css"> </head> <body> <section class="main-section"> <div class="container"> <?php if (isset($_SESSION['msg'])) : ?> <div class="toast-container position-fixed bottom-0 end-0 p-3"> <div class="toast fade show" role="alert" aria-live="assertive" aria-atomic="true"> <div class="toast-header <?php echo $_SESSION['class']; ?>"> <strong class="me-auto">Message</strong> <button type="button" class="btn-close text-white" data-bs-dismiss="toast" aria-label="Close"></button> </div> <div class="toast-body"> <?php $message = $_SESSION['msg']; unset($_SESSION['msg']); echo $message; ?> </div> </div> </div> <?php endif; ?> <div class="row justify-content-center align-items-center" style="height:100vh;"> <div class="col-md-7 col-lg-4"> <div class="box rounded"> <div class="img"></div> <div class="login-box p-5"> <h2 class="pb-4">Login</h2> <form action="" method="post"> <div class="mb-4"> <input type="email" class="form-control" placeholder="Enter Email address" name="email"> </div> <div class="mb-4"> <input type="password" class="form-control" placeholder="Enter Password" name="password"> </div> <div class="d-grid gap-2"> <button type="submit" class="btn btn-primary" name="login">Login</button> </div> </form> <div class="py-4 text-center"> Join now, <a href="signup.php" class="link">Sign Up</a> </div> </div> </div> </div> </div> </div> </section> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> </body> <!-- Coded by https://beproblemsolver.com Visit for more such code --> </html>
Below is the code for our signup page:
signup.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); require_once("function.php"); session_start(); if (isset($_POST['signup'])) { $name = santize($_POST['name']); $email = santize($_POST['email']); $inputpassword = santize($_POST['password']); $password = md5($inputpassword); $sql = "INSERT INTO users (name, email, password) VALUES ('$name', '$email', '$password');"; if (mysqli_query($conn, $sql)) { $_SESSION['msg'] = "You have Signed Up Successfully"; $_SESSION['class'] = "text-bg-success"; header("Location: index.php"); exit(); } else { $_SESSION['msg'] = "Sign Up failed"; $_SESSION['class'] = "text-bg-danger"; header("Location: index.php"); exit(); } } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz SignUp</title> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="css/style.css"> </head> <body> <section class="main-section"> <div class="container"> <div class="row justify-content-center align-items-center" style="height:100vh;"> <div class="col-md-7 col-lg-4"> <div class="box rounded"> <div class="img-2"></div> <div class="login-box p-5"> <h2 class="pb-4">Sign Up</h2> <form action="" method="post"> <div class="mb-4"> <input type="text" class="form-control" placeholder="Enter Name" name="name" required> </div> <div class="mb-4"> <input type="email" class="form-control" placeholder="Enter Email address" name="email" required> </div> <div class="mb-4"> <input type="password" class="form-control" placeholder="Enter Password" name="password" required> </div> <div class="d-grid gap-2"> <button type="submit" class="btn btn-primary-1" name="signup">Sign Up</button> </div> </form> <div class="py-4 text-center"> <a href="index.php" class="link">Login</a> </div> </div> </div> </div> </div> </div> </section> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> </body> <!-- Coded by https://beproblemsolver.com Visit for more such code --> </html>
Let’s take a break and enjoy 16 isekai with OP MC in 2024. Enjoy 😎😎!
Dashboard and Quiz Section
To create a dashboard for an online quiz system, we implement a set of features to manage the quiz questions and answers. Here are the steps to create a basic dashboard:
dashboard.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); session_start(); if (!isset($_SESSION['login_active'])) { header("Location: index.php"); exit(); } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz Dashboard</title> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="css/style.css"> </head> <body> <section class="main-section"> <nav class="navbar navbar-expand-lg bg-body-tertiary bg-dark" data-bs-theme="dark"> <div class="container-fluid"> <a class="navbar-brand" href="dashboard.php">Quiz</a> <button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav me-auto mb-2 mb-lg-0"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Link</a> </li> </ul> <div class="d-flex"> <a class="btn btn-danger" href="logout.php">Logout</a> </div> </div> </div> </nav> <div class="container"> <?php if (isset($_SESSION['msg'])) : ?> <div class="toast-container position-fixed bottom-0 end-0 p-3"> <div class="toast fade show" role="alert" aria-live="assertive" aria-atomic="true"> <div class="toast-header <?php echo $_SESSION['class']; ?>"> <strong class="me-auto">Success</strong> <button type="button" class="btn-close text-white" data-bs-dismiss="toast" aria-label="Close"></button> </div> <div class="toast-body"> <?php $message = $_SESSION['msg']; unset($_SESSION['msg']); echo $message; ?> </div> </div> </div> <?php endif; ?> <div class="row justify-content-center"> <h2 class="pt-4">Welcome to Dashboard, <?php echo $_SESSION['login_active']['0']; ?></h2> <div class="col-md-6"> <div class="card m-5 p-3"> <div class="card-body"> <h3 class="card-title py-2">Start taking Quiz</h3> <a href="quiz.php" class="btn btn-warning m-2">Start the Quiz</a> </div> </div> </div> </div> </div> </section> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> </body> <!-- Coded by https://beproblemsolver.com Visit for more such code --> </html>
We use an HTML form where users can take the online quiz system. Our form display each question along with multiple-choice options. Users can select one option for each question. Here is our PHP script:
quiz.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); require_once("function.php"); session_start(); if (!isset($_SESSION['login_active'])) { header("Location: index.php"); exit(); } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz</title> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="css/style.css"> </head> <body> <section class="main-section"> <nav class="navbar navbar-expand-lg bg-body-tertiary bg-dark" data-bs-theme="dark"> <div class="container-fluid"> <a class="navbar-brand" href="dashboard.php">Quiz</a> <button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav me-auto mb-2 mb-lg-0"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="dashboard.php">Dashboard</a> </li> </ul> <div class="d-flex"> <a class="btn btn-danger" href="logout.php">Logout</a> </div> </div> </div> </nav> <form action="checkanswers.php" method="post"> <?php for ($i = 1; $i <= totalquestion($conn); $i++) : $sql = "SELECT * FROM questions where qid = $i"; $result = mysqli_query($conn, $sql); ?> <div class="container"> <div class="row justify-content-center"> <?php while ($row = mysqli_fetch_assoc($result)) : $sql = "SELECT * FROM answers where ans_id = $i"; $result = mysqli_query($conn, $sql); ?> <div class="col-md-8"> <div class="card my-2 p-3"> <div class="card-body"> <h5 class="card-title py-2">Q.<?php echo $row['qid'] . " " . $row["question"];; ?> </h5> <?php while ($row = mysqli_fetch_assoc($result)) : ?> <div class="form-check"> <input type="radio" class="form-check-input" name="checkanswer[<?php echo $row['ans_id']; ?>]" value="<?php echo $row['aid']; ?>"> <?php echo $row['answer']; ?> </div> <?php endwhile ?> </div> </div> </div> <?php endwhile ?> <?php endfor ?> <div class="col-md-8 mb-5"> <button type="submit" class="btn btn-success" name="answer-submit">Submit Answers</button> </div> </div> </div> </form> </section> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> </body> <!-- Coded by https://beproblemsolver.com Visit for more such code --> </html>
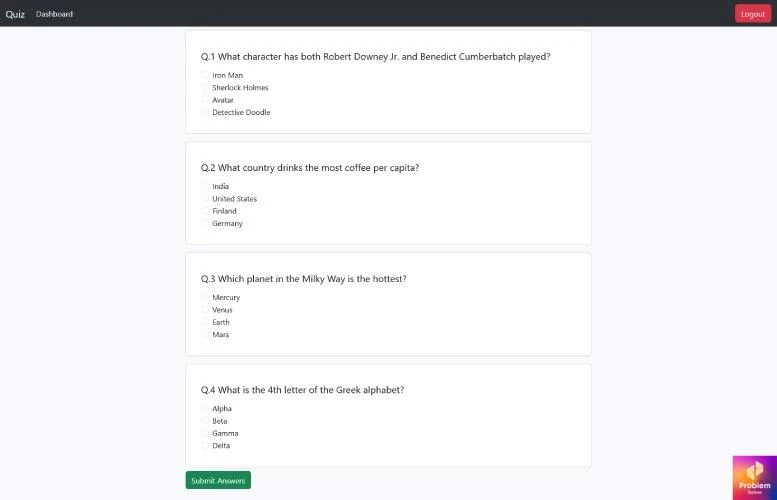
Logic Part of Online Quiz System
Create a PHP script to process the submitted quiz form. Retrieve the selected answers from the form and compare them with the correct answers stored in the database. Calculate the user’s score based on the number of correct answers.
checkanswers.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); session_start(); if (isset($_POST['answer-submit'])) { // Checking if our Questions are even attempted if (!empty($_POST['checkanswer'])) { // Set a flag for correct answers $correctAnswers = 0; $selected = $_POST['checkanswer']; $sql = "SELECT * FROM questions"; $result = mysqli_query($conn, $sql); $i = 1; while ($row = mysqli_fetch_assoc($result)) { // Matching Database Answerid with User selected answer id // If ans_id are matched our flag value is updated if ($row['ans_id'] == $selected[$i]) { $correctAnswers++; } $i++; } // Stored our score and attempted question value in session to be used on Result page $_SESSION['attempted'] = count($_POST['checkanswer']); $_SESSION['score'] = $correctAnswers; header("Location: result.php"); exit(); } else { // If Question not attempted set these variable like this $_SESSION['attempted'] = 0; $_SESSION['score'] = 0; header("Location: result.php"); exit(); } }
Finally, to display the result, we code our result.php page for the online quiz system PHP source code. You can also show the correct answers for each question if desired.
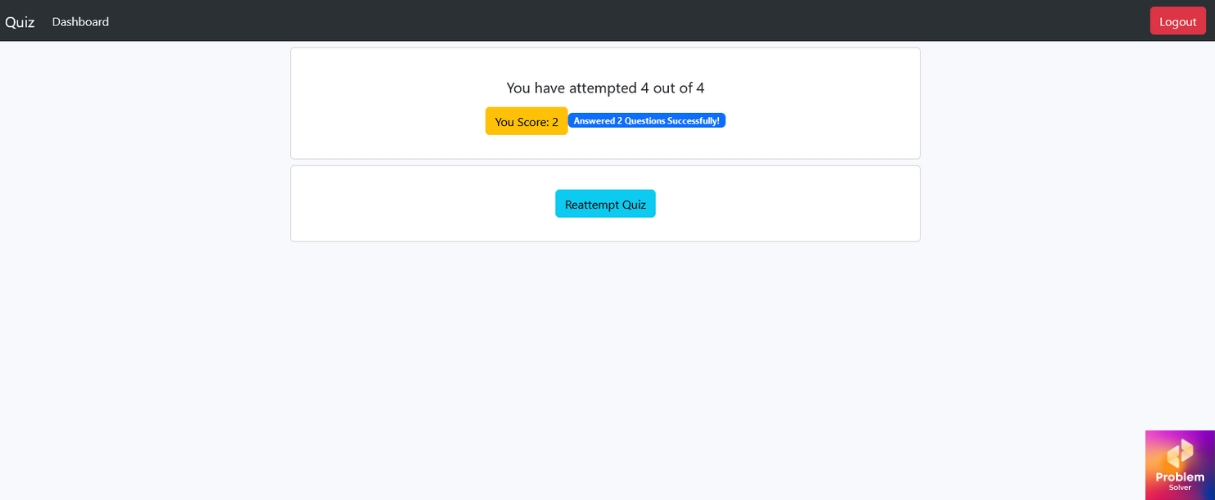
result.php
<!-- Coded by https://beproblemsolver.com Visit for more such code --> <?php require_once("connect.php"); require_once("function.php"); session_start(); if (!isset($_SESSION['login_active'])) { header("Location: index.php"); exit(); } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz</title> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="css/style.css"> </head> <body> <section class="main-section"> <nav class="navbar navbar-expand-lg bg-body-tertiary bg-dark" data-bs-theme="dark"> <div class="container-fluid"> <a class="navbar-brand" href="dashboard.php">Quiz</a> <button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav me-auto mb-2 mb-lg-0"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="dashboard.php">Dashboard</a> </li> </ul> <div class="d-flex"> <a class="btn btn-danger" href="logout.php">Logout</a> </div> </div> </div> </nav> <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <?php if ($_SESSION['score'] == 0) : ?> <div class="card my-2 p-3 text-center"> <div class="card-body"> <h5 class="card-title py-2 text-center">No Question Attempted</h5> <button class="btn btn-warning">You Score is : <?php echo $_SESSION['score']; ?></button> </div> </div> <?php else : ?> <div class="card my-2 p-3 text-center"> <div class="card-body"> <h5 class="card-title py-2 text-center">You have attempted <?php echo $_SESSION['attempted']; ?> out of <?php echo totalquestion($conn); ?></h5> <button class="btn btn-warning">You Score: <?php echo $_SESSION['score']; ?></button><span class="badge text-bg-primary">Answered <?php echo $_SESSION['score']; ?> Questions Successfully!</span> </div> </div> <?php endif ?> <div class="card my-2 p-3 text-center"> <div class="card-body"> <a class="btn btn-info" href="quiz.php">Reattempt Quiz</a> </div> </div> </div> </div> </div> </form> </section> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> </body> <!-- Coded by https://beproblemsolver.com Visit for more such code --> </html>
Download the full PHP multiple choice quiz source code from our GitHub link:
Conclusion
In conclusion, the Online Quiz System Project in PHP is a powerful tool for conducting quizzes online. It allows users to register, take quizzes and see their scores easily. With its simple and intuitive interface, PHP multiple choice Quiz Source code also makes it fun for PHP developers.
If you only want a PHP User Login system, check out this post. With its user-friendly interface and a whole bunch of extensions, you can download VS Code for your code projects too. Hope you liked it. Feel free to comment and share this article with friends and family.
Also if you have any doubts or improvements, do share them with us. Ta-da! Keep Coding guys and gals!
I have been struggling with this issue for a while and your post has provided me with much-needed guidance and clarity Thank you so much