Introduction
Do you want to develop an API with PHP, JSON, and POSTMAN?
Or are you simply looking for a way to build API for your Android App or IOS app?
Look no further!
In this tutorial, I will show you how to do just that!
We’ll go over the basics of each technology, we are using. Then dive into how to use them together to create API for your need. By the end of this tutorial, you’ll be able to create your API with PHP and JSON.
Before diving in, might I recommend a fantastic free editor that I love to use? Check out this post for more details.
What is an API?
So you want to build API or maybe multiple APIs?
First, let’s understand what is an API and why we rely on them in modern web app development.
The official definition you will find is: API stands for Application Programming Interface.
What does that mean?😑
In simple words, without techy jargon, an API is a piece of code you write, that allows two different pieces of software to communicate with each other.
API is just way to allow two different software to connect.
Why use API though? Simple. Have you ever used an android app where you are fetching and sending data most likely you have? Then you have already used an API.
Yeah! API is everywhere on the internet!🤯
With an API you connect databases like MySQL or MongoDB (or any other) to any frontend interface as an Android app or Web app. And foremost, APIs can be incredibly fast and easy to use.
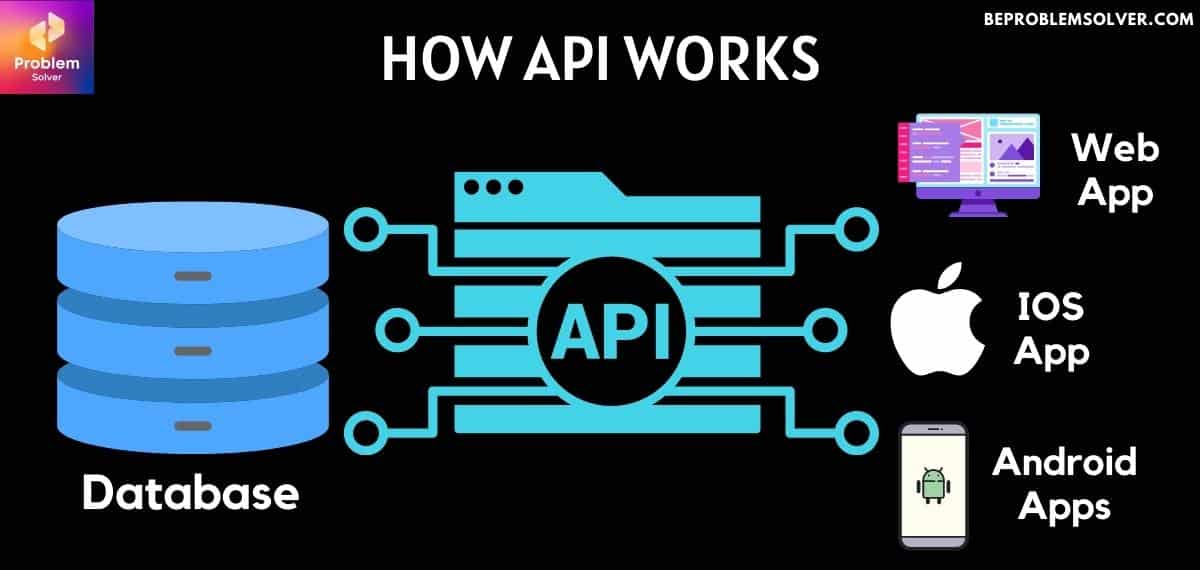
So you understand the basics of API and how it works behind the scene. Let’s jump into the technology we are going to use in our API build.
Why should you develop an API with PHP?
While there are many languages & frameworks for creating APIs, we are going to use PHP for this purpose.
But why?🤨
Let me answer the question in detail:
- PHP-based APIs are extremely easy to deploy. You don’t need to host the API on a separate physical server like Java or C. Which reduces overall cost.
- Despite being free, PHP is very powerful and you can use it to create very complex APIs.
- Even if you haven’t ever coded a programming language, PHP is easier to understand and learn. Remember HTML and CSS are structuring/styling languages, not programming ones.
So now you know why I chose PHP as the backbone of my API. Let’s look at other technology and tool we need to build API.
What is JSON?
If you ever worked with App Developer, as I have, you will love how much they appreciate the JSON.
Why?
I know I am very fond of why question. But it’s vital to understand the reason behind why JSON dominates the API building market. Let me explain:
- JSON, as you must know, stands for JavaScript Object Notation.
- JSON is not JavaScript. Please be careful not to confuse them.😡
- Yes, it looks similar to JavaScript objects but that’s because JSON shares a common codebase with ECMAScript, C++, and even C. But JSON is an independent and complete language in itself.
- It’s an ultra-lightweight data-interchange format.
- It’s language-independent, platform-independent, and most importantly, developer-friendly. The only language that comes close to competing is XML but it has its issues.
- JSON data can be written in any editor, sent over the network, and parsed by any platform. It may not seem that important in a modern environment where we have high-processing systems available. But in certain situations, JSON adaptability is a lifesaver. 😇
See how vital JSON is for our process to create API. Let’s jump to the last tool we need to get started.
What is POSTMAN?
Before you joke, no I am not talking about the Postman who delivers letters. 🤣
Our POSTMAN is an extremely powerful and necessary tool for testing and resolving bugs with our create API in PHP process.
So is it an absolute necessity? The answer would be No.
But other ways of testing your build API process are too time-consuming and not much accurate. POSTMAN is the tool all professionals rely upon.
In past, POSTMAN used to be a browser extension but now it’s a full-fledged software you can download. We only need the free version for our needs.
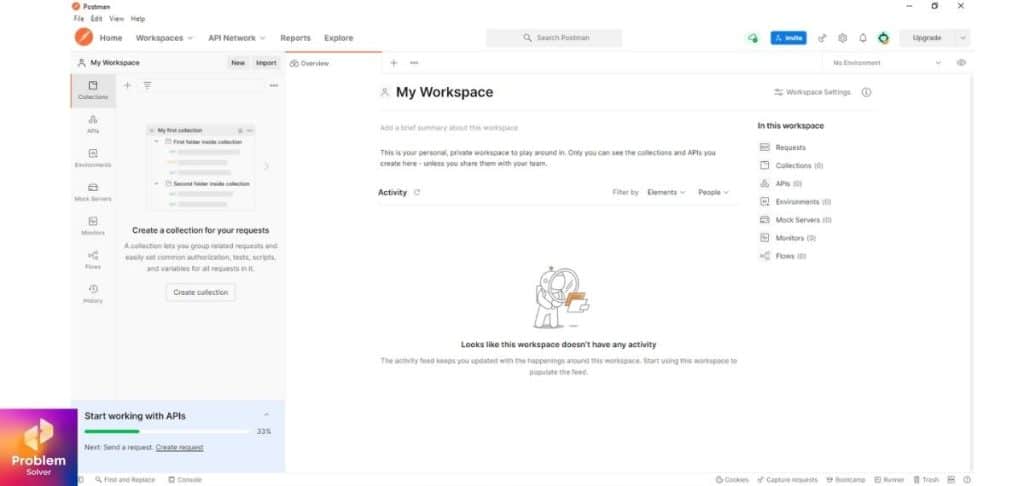
The process to develop an API
Now that we have acquainted with all the tools we will need. Let’s jump into the process of building API:
Establish Database
We build the database first. I will name my database “students“. Feel free to name your database anything. But keep a note of it as you will need it later on.
Next, we create a table “studentdata” which will hold our data. To make the table and its field quickly. Run the below SQL command:
CREATE TABLE `studentdata` ( `id` int(11) NOT NULL, `name` varchar(50) NOT NULL, `age` int(5) NOT NULL, `city` varchar(50) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
If you don’t know how to run SQL commands in PHPMyAdmin. Read my previous article, I’ve explained there how to run SQL.
Now your database has a table and looks like this:

Provide Data in Database
Now that we have a database and a table let us insert some data by SQL for now. Don’t worry we will create a process in PHP to insert data through our API. But that comes later.
INSERT INTO `studentdata` (`id`, `name`, `age`, `city`) VALUES (2, 'Suresh', 26, 'Chennai'), (3, 'Abhimanyu', 19, 'Kolkata'), (4, 'Raj', 25, 'Kanpur'), (5, 'Subash', 27, 'Bhopal'), (7, 'Amir', 35, 'Imphal');
For now, we have some data in our database that we can interact with, when we build our API.
Connect Database with PHP
Now we connect to our database with PHP. For this purpose, I created the “connect.php” file. Remember to PHP create API JSON, we need a database to handle our data.
Note: “.php” is our extension for PHP files.
<?php $conn = mysqli_connect('localhost', 'root', '', 'students'); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } ?>
Build API – GET Request
Now we start coding with PHP. So bring your favorite code editor as I started up with mine. Let’s write the first API file that will fetch everything from our database.
Filename: “api_fetch_all.php“.
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); include 'connect.php'; $sql = "select * from studentdata"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { //mysqli_fetch_all gives us the data in 2D array format. // It's second parameter decide whether its assoc array or indexed. Or maybe both $data = mysqli_fetch_all($result, MYSQLI_ASSOC); echo json_encode($data); } else { echo json_encode(['msg' => 'No Data!', 'status' => false]); } ?>
Let me explain what we wrote and why:
- Our first line “header(‘Content-Type: application/json’)“ is telling our file that we are using the JSON data format to exchange data. Without this line of code, our API will never work.
- The next header line is simply allowing our API file to access data. Sometimes you will see developers skip this line. And sometimes that’s okay. But for the best result and to avoid any future error, we did define it.
- Now, we ran the standard PHP code to select all data from the database. Then fetched that data and stored it in our “$data” variable.
- Using the famous PHP method “json_encode()“, we convert our data into JSON format. And “echo” this JSON data.
- Lastly, we encoded an error msg and status, just in case our data failed to be obtained.
If you have reached this point. Congratulations! 😎
You are halfway done with learning the basics of how to create API in PHP. We have also built our first API file so let us test it with POSTMAN.
Test API with POSTMAN
Testing API with POSTMAN might seem daunting at first. But no worries, I will guide you through that process.
- Open your POSTMAN app and click the “+” icon where it says “Untitled Request“.
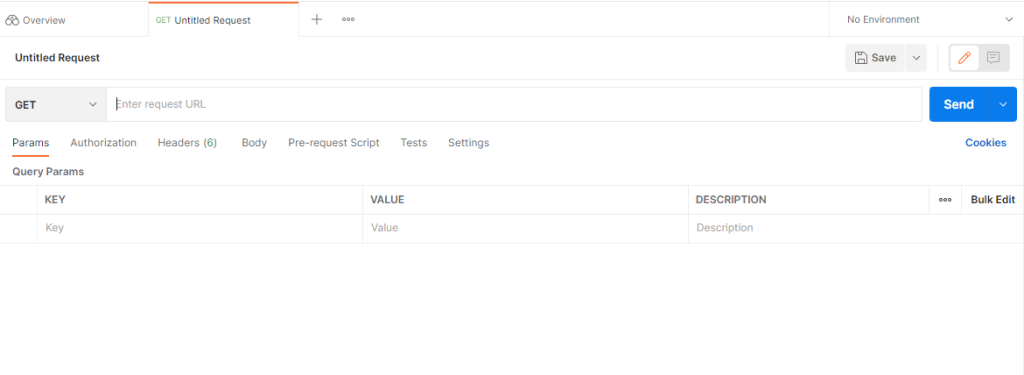
- Enter your API URL in the “URL-send” and set it to “GET”
- In the lower tab choose the header section. Enter header to content-type: application/JSON. Then hit Enter button.
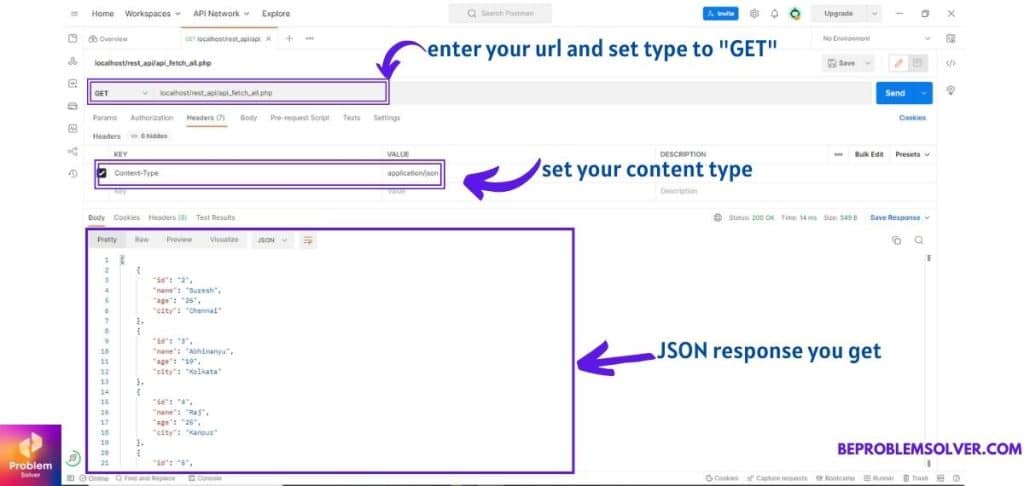
- If done everything, you will see the JSON response in the downward part.
Now we have completed our first API. This API will fetch all data for us from the database in JSON format. Let’s continue the rest of the process of developing an API.
Create API – GET Single data
Now let’s code our API file which will fetch single data by id as reference.
Filename: api_fetch_single.php
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); // This is the data which is being sent to request a single data. // Since we know this request will be in json format we must decode before passing it into our php logic // file_get_contents is reading file into a string. // "php://input" is special key which insure that we can get any format of data. Even if its raw data. // True argument of json decode makes sure that we get response in assoc array $data = json_decode(file_get_contents("php://input"), true); // we are getting our passed data in $data array. But to ensure securty we will change name of id to sid. Remember we are being requested data on basis of sid after all. $student_id = $data['sid']; include 'connect.php'; $sql = "select * from studentdata where id = $student_id"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { //mysqli_fetch_all gives us the data in 2D array format. // It's second parameter decide whether its assoc array or indexed. Or maybe both $data = mysqli_fetch_all($result, MYSQLI_ASSOC); echo json_encode($data); } else { echo json_encode(['msg' => 'No Data!', 'status' => false]); } ?>
Unlike the previous API where we were fetching every data in the table, now we are targeting a specific one.
Also, we provide code “file_get_contents” which allows our reading file into a string. And “php://input” is a special key that ensures that we can get any format of data. Even if it’s raw data.
Similarly, we will create API files for POST and DELETE requests too.
Develop an API – POST Request for Insert & Update
First, let’s learn how to build a POST request API that will insert our data into the database.
Filename: api_insert.php
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Methods: POST'); header('Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Methods, Access-Control-Allow-Headers, Authorization, X-Requested-With'); // Since we are inserting data we pass two extra headers. // 1st allow us to set the method of insert. i.e. POST in rest api // 2nd determines which type of headers can be sent. It's a secuirty header. // 'Authorization' is set for authorizing insert data. While 'X-Requested-With' is set for passing data as json $data = json_decode(file_get_contents("php://input"), true); $sname = $data['sname']; $sage = $data['sage']; $scity = $data['scity']; include 'connect.php'; $sql = "insert into studentdata (name, age, city) values ('$sname', '$sage', '$scity')"; if (mysqli_query($conn, $sql)) { echo json_encode(['msg' => 'Data Inserted Successfully!', 'status' => true]); } else { echo json_encode(['msg' => 'Data Failed to be Inserted!', 'status' => false]); } ?>
Next, we are up for Update Requests. Which again will be done with POST Request but with little modifications. 🧐
Filename: api_update.php
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Methods: PUT'); header('Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Methods, Access-Control-Allow-Headers, Authorization, X-Requested-With'); // To update is similar to insert. Except in rest api update request is done throough PUT method $data = json_decode(file_get_contents("php://input"), true); $sid = $data['sid']; $sname = $data['sname']; $sage = $data['sage']; $scity = $data['scity']; include 'connect.php'; $sql = "update studentdata set name = '$sname', age = '$sage', city = '$scity' where id = '$sid'"; if (mysqli_query($conn, $sql)) { echo json_encode(['msg' => 'Data Updated Successfully!', 'status' => true]); } else { echo json_encode(['msg' => 'Data Failed to be Updated!', 'status' => false]); } ?>
Remember while updating, we are providing a specific id with our “update-data“.
Last we have only the Delete and Search API part left. Let’s keep going …💻
Build API – DELETE Request
When writing Delete Request, we add two new headers type.
- header(‘Access-Control-Allow-Methods: DELETE’) – allow us to set our API to delete files as needed. Without it, you will run into errors.
- Next headers allow us to give more control of files to API queries. It’s recommended to add them.
Filename: api_delete.php
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Methods: DELETE'); header('Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Methods, Access-Control-Allow-Headers, Authorization, X-Requested-With'); // To delete in rest api, we use DELETE method $data = json_decode(file_get_contents("php://input"), true); $sid = $data['sid']; include 'connect.php'; $sql = "delete from studentdata where id = '$sid'"; if (mysqli_query($conn, $sql)) { echo json_encode(['msg' => 'Data Deleted Successfully!', 'status' => true]); } else { echo json_encode(['msg' => 'Data Failed to be Deleted!', 'status' => false]); } ?>
Before finalizing, do test your API with POSTMAN.
And now let’s move to the last API file of the day. It’s quite an important one though, so stay alert. Also if you want to link your HTML form to Google Spreadsheet, then check out this post.
Search API – GET Request
Filename: api_search.php
<?php header('Content-Type: application/json'); header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Methods: POST'); $data = json_decode(file_get_contents("php://input"), true); $searchterm = $data['search']; include 'connect.php'; $sql = "select * from studentdata where name like '%$searchterm%'"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { $data = mysqli_fetch_all($result, MYSQLI_ASSOC); echo json_encode($data); } else { echo json_encode(['msg' => 'No Data Found to search query!', 'status' => false]); } ?>
See what your search should look like in the below image:
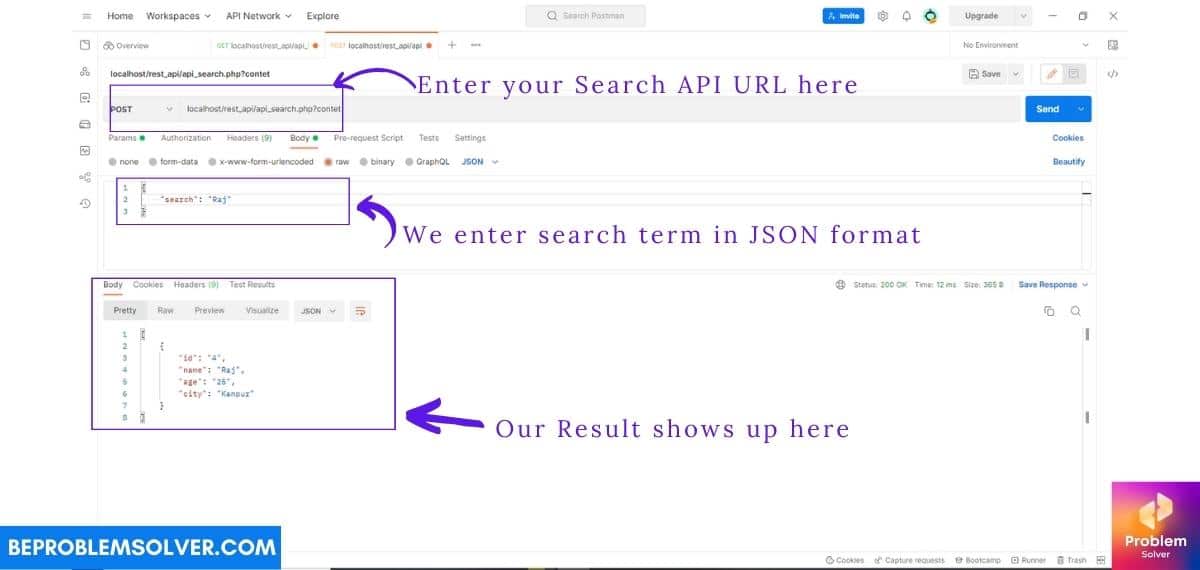
Note: Search functionality in an API can be developed by either GET or POST request method. In GET method case we pass our search values to the URL. In the POST method, we use the normal JSON input. So you see there is nothing different about a PHP create API JSON.
Conclusion
So there you have it! 🥳
A quick and easy guide on how to develop an API with PHP, JSON, and POSTMAN. I hope this has been helpful for you. With this detailed guide at your side, we at Be Problem Solver believe you feel more confident to develop APIs.
In our next posts, we show you how to use this API and other third-party APIs in projects.
If you have any questions or comments, then feel free to reach out. And do check out my other post about adding jazz to your website with page scroll animations.
Ta-da! Guys and Gals! Happy coding!
Very good article! We will be linking to this great post on our website.
Keep up the good writing.
This is by far the best writing on how to develop an API and test it using Postman, nothing on the net beats it.
I was almost giving up on learning API development.
Thank you very much, ready to support your works, thank you once again.
Thank you for your kind feedback.
I do trust all the ideas you’ve offered on your post.
They’re very convincing and can certainly work.
Still, the posts are very brief for starters. May just you please extend them a little
from next time? Thanks for the post.